This lesson teaches you to
- Add Pages to a Notification
When you'd like to provide more information without requiring users to open your app on their handheld device, you can add one or more pages to the notification on the wearable. The additional pages appear immediately to the right of the main notification card.
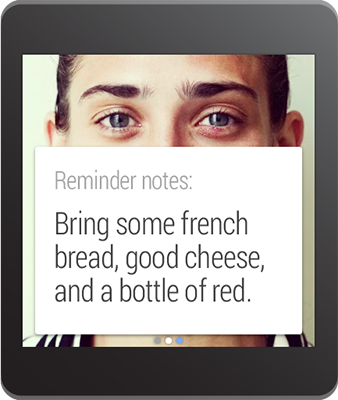
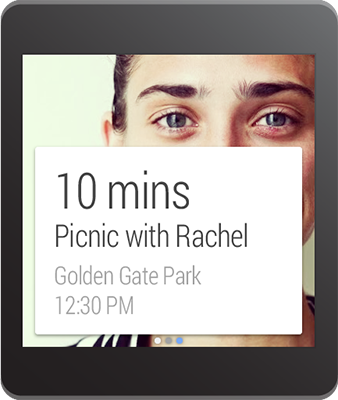
To create a notification with multiple pages:
- Create the main notification (the first page) with
NotificationCompat.Builder
, in the way you'd like the notification to appear on a handset. - Create the additional pages for the wearable with
NotificationCompat.Builder
. - Apply the pages to the main notification with the
addPage()
method or add multiple pages in aCollection
with theaddPages()
method.
For example, here's some code that adds a second page to a notification:
// Create builder for the main notification NotificationCompat.Builder notificationBuilder = new NotificationCompat.Builder(this) .setSmallIcon(R.drawable.new_message) .setContentTitle("Page 1") .setContentText("Short message") .setContentIntent(viewPendingIntent); // Create a big text style for the second page BigTextStyle secondPageStyle = new NotificationCompat.BigTextStyle(); secondPageStyle.setBigContentTitle("Page 2") .bigText("A lot of text..."); // Create second page notification Notification secondPageNotification = new NotificationCompat.Builder(this) .setStyle(secondPageStyle) .build(); // Extend the notification builder with the second page Notification notification = notificationBuilder .extend(new NotificationCompat.WearableExtender() .addPage(secondPageNotification)) .build(); // Issue the notification notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(notificationId, notification);