If you're unfamiliar with using Android Studio and the IntelliJ IDEA interface, this page provides some tips to help you get started with some of the most common tasks and productivity enhancements.
Productivity Features
Android Studio includes a number of features to help you be more productive in your coding. This section notes a few of the key features to help you work quickly and efficiently.
Smart Rendering
With smart rendering, Android Studio displays links for quick fixes to rendering errors. For example, if you add a button to the layout without specifying the width and height atttributes, Android Studio displays the rendering message Automatically add all missing attributs. Clicking the message adds the missing attributes to the layout.
Bitmap rendering in the debugger
While debugging, you can now right-click on bitmap variables in your app and invoke View Bitmap. This fetches the associated data from the debugged process and renders the bitmap in the debugger.
Figure 1. Bitmap Rendering
Output window message filtering
When checking build results, you can filter messages by message type to quickly locate messages of interest.
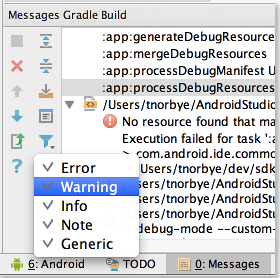
Figure 2. Filter Build Messages
Hierarchical parent setting
The activity parent can now be set in the Activity Wizard when creating a new
activity. Setting a hierarchal parent sets the Up
button to automatically
appear in the app's Action bar when viewing a child activity, so the Up
button no longer needs to be manually specified in the menu.xml file.
Creating layouts
Android Studio offers an advanced layout editor that allows you to drag-and-drop widgets into your layout and preview your layout while editing the XML.
While editing in the Text view, you can preview the layout on devices by opening the Preview pane available on the right side of the window. Within the Preview pane, you can modify the preview by changing various options at the top of the pane, including the preview device, layout theme, platform version and more. To preview the layout on multiple devices simultaneously, select Preview All Screen Sizes from the device drop-down.
Figure 3. Preview All Screens
You can switch to the graphical editor by clicking Design at the bottom of the window. While editing in the Design view, you can show and hide the widgets available to drag-and-drop by clicking Palette on the left side of the window. Clicking Designer on the right side of the window reveals a panel with a layout hierarchy and a list of properties for each view in the layout.
Working with IntelliJ
This section list just a few of the code editing practices you should consider using when creating Android Studio apps.
For complete user documentation for the IntelliJ IDEA interface (upon which Android Studio is based), refer to the IntelliJ IDEA documentation.
Alt + Enter key binding
For quick fixes to coding errors, the IntelliJ powered IDE implements the Alt + Enter key binding to fix errors (missing imports, variable assignments, missing references, etc) when possible, and if not, suggest the most probable solution.
Ctrl + D key binding
The Ctrl + D key binding is great for quickly duplicating code lines or fragments. Simply select the desired line or fragment and enter this key binding.
Navigate menu
In case you're not familiar with an API class, file or symbol, the Navigate menu lets you jump directly to the class of a method or field name without having to search through individual classes.
Inspection scopes
Scopes set the color of code segments for easy code identification and location. For example, you can set a scope to identify all code related to a specific action bar.
External annotations
Specify annotations within the code or from an external annotation file. The Android Studio IDE keeps track of the restrictions and validates compliance, for example setting the data type of a string as not null.
Injecting languages
With language injection, the Android Studio IDE allows you to work with islands of different languages embedded in the source code. This extends the syntax, error highlighting and coding assistance to the embedded language. This can be especially useful for checking regular expression values inline, and validating XML and SQL statments.
Code folding
This allows you to selectively hide and display sections of the code for readability. For example, resource expressions or code for a nested class can be folded or hidden in to one line to make the outer class structure easier to read. The inner clas can be later expanded for updates.
Image and color preview
When referencing images and icons in your code, a preview of the image or icon appears
(in actual size at different densities) in the code margin to help you verify the image or icon
reference. Pressing F1
with the preview image or icon selected displays resource asset
details, such as the dp settings.
Quick F1 documentation
You can now inspect theme attributes using View > Quick Documentation (F1), see the theme inheritance hierarchy, and resolve values for the various attributes.
If you invoke View > Quick Documentation (usually bound to F1) on the theme attribute ?android:textAppearanceLarge, you will see the theme inheritance hierarchy and resolved values for the various attributes that are pulled in.
New Allocation Tracker integration in the Android/DDMS window
You can now inspect theme attributes using View > Quick Documentation
F1
, see the theme inheritance hierarchy, and resolved values for the
various attributes.
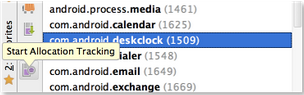
Figure 4. Allocation Tracker
Keyboard Commands
The following tables list keyboard shortcuts for common operations.
Note: This section lists Android Studio keyboard shortcuts for the default keymap. To change the default keymap on Windows and Linux, go to File > Settings > Keymap. If you're using Mac OS X, update your keymap to use the Mac OS X 10.5+ version keymaps under Android Studio > Preferences > Keymap.
Table 1. Programming key commands
Action | Android Studio Key Command |
---|---|
Command look-up (autocomplete command name) | CTRL + SHIFT + A |
Project quick fix | ALT + ENTER |
Reformat code | CTRL + ALT + L (Win) OPTION + CMD + L (Mac) |
Show docs for selected API | CTRL + Q (Win) F1 (Mac) |
Show parameters for selected method | CTRL + P |
Generate method | ALT + Insert (Win) CMD + N (Mac) |
Jump to source | F4 (Win) CMD + down-arrow (Mac) |
Delete line | CTRL + Y (Win) CMD + Backspace (Mac) |
Search by symbol name | CTRL + ALT + SHIFT + N (Win) OPTION + CMD + O (Mac) |
Table 2. Project and editor key commands
Action | Android Studio Key Command |
---|---|
Build | CTRL + F9 (Win) CMD + F9 (Mac) |
Build and run | SHIFT + F10 (Win) CTRL + R (Mac) |
Toggle project visibility | ALT + 1 (Win) CMD + 1 (Mac) |
Navigate open tabs | ALT + left-arrow; ALT + right-arrow (Win) CTRL + left-arrow; CTRL + right-arrow (Mac) |
For a complete keymap reference guide, see the IntelliJ IDEA documentation.