How you setup the Android Support Libraries in your development project depends on what features you want to use and what range of Android platform versions you want to support with your application.
This document guides you through downloading the Support Library package and adding libraries to your development environment.
Downloading the Support Libraries
The Android Support Library package is provided as a supplemental download to the Android SDK and is available through the Android SDK Manager. Follow the instructions below to obtain the Support Library files.
To download the Support Library through the SDK Manager:
- Start the Android SDK Manager.
- In the SDK Manager window, scroll to the end of the Packages list, find the Extras folder and, if necessary, expand to show its contents.
- Select the Android Support Library item.
Note: If you're developing with Android Studio, select and install the Android Support Repository item instead.
- Click the Install packages... button.
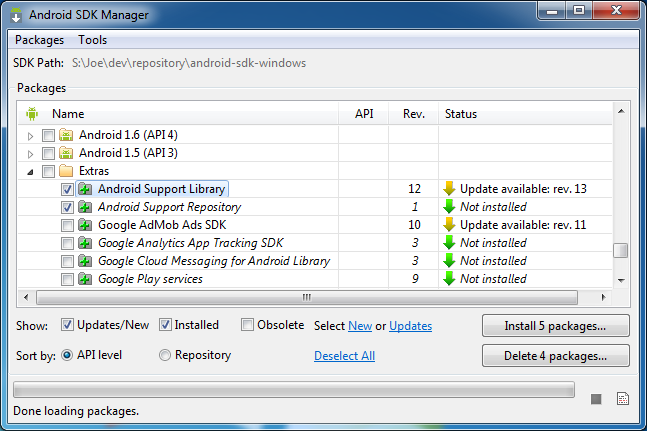
Figure 1. The Android SDK Manager with the Android Support Library selected.
After downloading, the tool installs the Support Library files to your existing Android SDK
directory. The library files are located in the following subdirectory of your SDK:
<sdk>/extras/android/support/
directory.
Choosing Support Libraries
Before adding a Support Library to your application, decide what features you want to include and the lowest Android versions you want to support. For more information on the features provided by the different libraries, see Support Library Features.
Adding Support Libraries
In order to use a Support Library, you must modify your application's project's classpath dependencies within your development environment. You must perform this procedure for each Support Library you want to use.
Some Support Libraries contain resources beyond compiled code classes, such as images or XML files. For example, the v7 appcompat and v7 gridlayout libraries include resources.
If you are not sure if a library contains resources, check the Support Library Features page. The following sections describe how to add a Support Library with or without resources to your application project.
Adding libraries without resources
To add a Support Library without resources to your application project:
- Make sure you have downloaded the Android Support Library using the SDK Manager.
- Create a
libs/
directory in the root of your application project. - Copy the JAR file from your Android SDK installation directory (e.g.,
<sdk>/extras/android/support/v4/android-support-v4.jar
) into your application's projectlibs/
directory. - Right click the JAR file and select Build Path > Add to Build Path.
- Make sure you have downloaded the Android Support Repository using the SDK Manager.
- Open the
build.gradle
file for your application. - Add the support library to the
dependencies
section. For example, to add the v4 support library, add the following lines:dependencies { ... compile "com.android.support:support-v4:18.0.+" }
Adding libraries with resources
To add a Support Library with resources (such as v7 appcompat for action bar) to your application project:
Create a library project based on the support library code:
- Make sure you have downloaded the Android Support Library using the SDK Manager.
- Create a library project and ensure the required JAR files are included in the project's
build path:
- Select File > Import.
- Select Existing Android Code Into Workspace and click Next.
- Browse to the SDK installation directory and then to the Support Library folder.
For example, if you are adding the
appcompat
project, browse to<sdk>/extras/android/support/v7/appcompat/
. - Click Finish to import the project. For the v7 appcompat project, you should now see a new project titled android-support-v7-appcompat.
- In the new library project, expand the
libs/
folder, right-click each.jar
file and select Build Path > Add to Build Path. For example, when creating the the v7 appcompat project, add both theandroid-support-v4.jar
andandroid-support-v7-appcompat.jar
files to the build path. - Right-click the library project folder and select Build Path > Configure Build Path.
- In the Order and Export tab, check the
.jar
files you just added to the build path, so they are available to projects that depend on this library project. For example, theappcompat
project requires you to export both theandroid-support-v4.jar
andandroid-support-v7-appcompat.jar
files. - Uncheck Android Dependencies.
- Click OK to complete the changes.
You now have a library project for your selected Support Library that you can use with one or more application projects.
Add the library to your application project:
- In the Project Explorer, right-click your project and select Properties.
- In the category panel on the left side of the dialog, select Android.
- In the Library pane, click the Add button.
- Select the library project and click OK. For example, the
appcompat
project should be listed as android-support-v7-appcompat. - In the properties window, click OK.
Note: If you are using the android-support-v7-mediarouter
support
library, you should note that it depends on the android-support-v7-appcompat
library.
In order for the v7 mediarouter library to compile, you must import both library projects into
your development workspace. Then follow the procedure above to add the v7 appcompat project as a
library to the v7 mediarouter library project.
- Make sure you have downloaded the Android Support Repository using the SDK Manager.
- Open the
build.gradle
file for your application. - Add the support library feature project identifier to the
dependencies
section. For example, to include theappcompat
project addcompile "com.android.support:appcompat-v7:18.0.+"
to the dependencies section, as shown in the following example:dependencies { ... compile "com.android.support:appcompat-v7:18.0.+" }
Using Support Library APIs
Support Library classes that provide support for existing framework APIs typically have the
same name as framework class but are located in the android.support
class packages,
or have a *Compat
suffix.
Caution: When using classes from the Support Library, be certain you import
the class from the appropriate package. For example, when applying the ActionBar
class:
android.support.v7.app.ActionBar
when using the Support Library.android.app.ActionBar
when developing only for API level 11 or higher.
Note: After including the Support Library in your application project, we strongly recommend using the ProGuard tool to prepare your application APK for release. In addition to protecting your source code, the ProGuard tool also removes unused classes from any libraries you include in your application, which keeps the download size of your application as small as possible. For more information, see ProGuard.
Further guidance for using some Support Library features is provided in the Android developer
training classes,
guides
and samples. For more information about the individual Support Library classes and methods, see
the android.support
packages in the API reference.
Manifest Declaration Changes
If you are increasing the backward compatibility of your existing application to an earlier
version of the Android API with the Support Library, make sure to update your application's
manifest. Specifically, you should update the android:minSdkVersion
element of the
<uses-sdk>
tag in the manifest to the new, lower version number, as
shown below:
<uses-sdk android:minSdkVersion="7" android:targetSdkVersion="17" />
The manifest setting tells Google Play that your application can be installed on devices with Android 2.1 (API level 7) and higher.
If you are using Gradle build files, the minSdkVersion
setting in the build file
overrides the manifest settings.
apply plugin: 'com.android.application' android { ... defaultConfig { minSdkVersion 8 ... } ... }
In this case, the build file setting tells Google Play that the default build variant of your application can be installed on devices with Android 2.2 (API level 8) and higher. For more information about build variants, see Build System Overview.
Note: If you are including the v4 support and v7 appcompat libraries in your
application, you should specify a minimum SDK version of "7"
(and not
"4"
). The highest support library level you include in your application determines
the lowest API version in which it can operate.
Code Samples
Each Support Library includes code samples to help you get started using the support APIs. The code is included in the download from the SDK Manager and is placed inside the Android SDK installation directory, as listed below:
- 4v Samples:
<sdk>/extras/android/support/samples/Support4Demos/
- 7v Samples:
<sdk>/extras/android/support/samples/Support7Demos/
- 13v Samples:
<sdk>/extras/android/support/samples/Support13Demos/
- App Navigation:
<sdk>/extras/android/support/samples/SupportAppNavigation/